A friend needs help....
Author |
Message |
lionheart
|
Posted: Tue Dec 13, 2005 9:53 pm Post subject: A friend needs help.... |
|
|
well....he needs helping creating a Hang man game..
Part 1:
Write methods to draw the hanging man as he looks after each guess. In other words, you should draw six pictures, with each picture incorporating the previous one. (Hint: Start with a DrawHat/drawOne () method, then let the DrawHead() method call it, etc.) Each method should call the method defined before it.)
You should modularize your code appropriately - following the design discussed in class would be a good start.
We will divide the source code for the hangman program into two classes. One will contain the main method, and one will contain the methods to handle the output of the program.
1. Begin a new project called Hangman. This program will contain your main method and eventually will include the code to play the game of hangman.
2. Type in the following:
/**
* Write a description of class Hangman here.
*
* @author (your name)
* @version (a version number or a date)
*/
public class Hangman
{
public static void main(String[ ] args)
{
c = new Console ( );
}
}
3. Click on New Class and name the new class GameOutput.
4. Delete all of the code in the new class except for the following:
/**
* Write a description of class GameOutput here.
*
* @author (your name)
* @version (a version number or a date)
*/
class GameOutput
{
}
5. One component of the game of hangman is the various stages of the hangman. There are seven different stages of the scaffold (empty; head only; head and body; head, body and one arm; and so on). Define the seven methods drawZero, drawOne, drawTwo, drawThree, drawFour, drawFive, and drawSix. The description for the first method is:
void drawZero( )
// Draws the hangman scaffold with zero body parts
// Output: The hangman scaffold
{
}
6. You now need to write the actual code for each of the methods that you defined in the class GameOutput. An easy way to produce these seven drawings is to decide how you want your entire hangman to look and to draw it on the screen using output statements. Once the final hangman is drawn to your satisfaction, use your editor to make a copy of the code and paste the copy in the method drawSix ( ). Now remove one of the legs from your drawing, copy, and then paste this copy in the method drawFive( ). Repeat this process until you arrive at the empty scaffold stage.
HINT: To output the backslash character, you must use the following code:
System.out.println ("\\");
7. Add the line
GameOutput game = new GameOutput ( );
under the line
c = new Console ( );
in your main method.
8. Put the lines in your main method so that it will call each of the methods in GameOutput. Add code that will allow the output to pause between each stage of the drawing.
9. Compile and run the program to make sure the method calls are operating as expected.
10. Add the method printDirections to the class GameOutput. You may use the directions as stated in the beginning of this lab, or you may rewrite them, but make sure the directions are complete so that the user knows how to play the game. Add a call to this new method before the calls to printing out the hangman.
11. Once a person has played your game, they probably will not want to see the directions every time the program is run. Modify the main method so that the user is asked whether to display the directions. If the answer is 'Y' or 'y' for "Yes", print the directions. Otherwise, do not print the directions.
12. In the class GameOutput add the method drawHangman with the following specification:
void drawHangman (int i)
// Draws the hangman picture corresponding to the input number of body part to be drawn
// Input: Number of body parts to draw
// Output: None
13. Use the switch statement to make the selection of the draw method. Test this method by calling it from your program seven times, once with each of the input value 0 through 6. Pause the output between each of these calls. Delete the lines with the calls to the individual methods drawZero ( ) etc.
Coding
"¢ The seven methods drawZero, drawOne, drawTwo, drawThree, drawFour, drawFive, and drawSix are written correctly.
"¢ The program calls the method drawHangman with the numbers 0 through 6.
"¢ The method drawHangman correctly uses the switch statement to draw each of the seven stages.
"¢ It correctly compares the letters and outputs the letter in correct spot.
Part 2:
The word file must be stored in dictionary.txt in a text file (10 four-letter words). Once these words are accessed, it must be stored in the program folder P2. The program must randomly choose one of these words to be the new hidden word.
Have your program allow the user to play the game of Hangman for the hidden word stored as an array of characters. Design your program so that the word could easily be changed without having to change any code - other than the statement declaring the word.
(Hint: use two parallel arrays: one to store the word and another to store the portion of the word that has been guessed.)
After each guess, you should show as much of the word as has been guessed and the appropriate drawing.
Notes:
1. You should modularize your code appropriately.
2. User guess should not be case-sensitive. That is, if they user enters 'A' or 'a' it should be a good guess if the word contains any occurrences of the letter 'a'.
3. If the user guesses the same wrong letter over and over, count each as a wrong guess and terminate the game after six wrong guesses.
4. If the user keeps guessing the same correct letter, output a warning only, do not count each as a wrong guess.
Design:
For this program, implement the following methods and use them in your solution for playing the game of hangman. Your main method will drive the game playing by calling the methods below (some of which will call each other, as needed). (You may add additional methods, but do not eliminate any of those described below.)
The following are methods for drawing the body in pieces; each generates the hanged man up to that part: drawOne () calls drawZero () before drawing the head, so the result is a head with a rope, etc. You can design your own seven drawing methods, rather than using those 7 described next as long as each calls the others before it, as described in the Part 1 above.
public void drawZero () //Scaffold or a rope
public void drawOne () // drawHead
public void drawTwo () // drawTorso
public void drawThree ()//drawLeftArm
public void drawFour ()//drawRightArm
public void drawFive ()//drawLeftLeg
public void drawSix ()//drawRightLeg
The following methods are useful for controlling the game.
1. public String initializeWord()
This initializes the "secret word" for playing the game. It retrieves the data files and returns a random word. Counts the space for the dashes..
(e.g., String secretWord;
secretWord = initializeWord(); )
2. public char getUserInput()
This prompts for user's guess.
3. Verify that guess is valid, by calling routine
isInputValid()
public boolean isInputValid(char input)
This verifies that input provided by user is a letter.
4. public boolean isGuessCorrect(char guess, String secretWord, char guessedWord[])
This compares if user's guess is correct - is this letter in the secret word? If so, it updates the guessed word.
// create the guessed word
guessedWord = new char [secretWord.length ()];
initCharArray (guessedWord, '-');
// initialize an array arr with ch
private static void initCharArray (char[] arr, char ch)
{
for (int i = 0 ; i < arr.length ; i++)
arr [i] = ch;
}
5. public void drawHangman(int whichWrongGuess)
This draws hangman if guess was not correct. Method drawHangman() calls appropriate drawing method (above) based on number of wrong guesses thus far.
6. public boolean isGameOver(int wrongGuesses, char secretWord[], char guessedWord[])
This checks if game is now over. Game is over if 6 wrong guesses have been entered or if the word has been guessed correctly.
14. Put your name, date, and a brief description of the project in comments at the top.
15. Attach the files Hangman.java, GameOutput.java, dictionary.txt and submit all in a folder called P2 to your instructor for a grade.
... lol uhm well he wants to talk to someone on msn..
so he can ask for help |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
wtd
|
Posted: Tue Dec 13, 2005 9:54 pm Post subject: (No subject) |
|
|
Try using code or syntax tags. |
|
|
|
|
 |
lionheart
|
Posted: Tue Dec 13, 2005 9:57 pm Post subject: uh.. |
|
|
nvm about that msn part..
talk here. |
|
|
|
|
 |
Tony
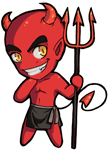
|
Posted: Wed Dec 14, 2005 9:16 am Post subject: (No subject) |
|
|
seem to be a set of instructions on how to write a program... with steps as specific as
Quote:
7. Add the line
GameOutput game = new GameOutput ( );
Quote:
9. Compile and run the program to make sure the method calls are operating as expected.
Which steps are actually problematic? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
codemage

|
Posted: Wed Dec 14, 2005 9:20 am Post subject: (No subject) |
|
|
Heh. That's a pretty accurate definition of an assignment given to "a friend of yours."
This "guy you know" should probably have some code done already so he can ask specific questions. |
|
|
|
|
 |
md

|
Posted: Wed Dec 14, 2005 2:29 pm Post subject: (No subject) |
|
|
Seems an aweful lot like an assignment you want someone to do for you... |
|
|
|
|
 |
wtd
|
Posted: Wed Dec 14, 2005 2:33 pm Post subject: (No subject) |
|
|
I suspected much the same when I tried to help and there was no interest in anything other than the answer. |
|
|
|
|
 |
|
|