What's False and What's Not?
Author |
Message |
wtd
|
Posted: Sun Nov 13, 2005 2:18 pm Post subject: What's False and What's Not? |
|
|
Ok, I'll come right out and say it. In Ruby, only two values are false.
and
Everything else is true.
For instance, a C programmer might have a conditional like...
code: | if (input_number % 2)
{
printf("%d is odd.\n", input_number);
}
else
{
printf("%d is even.\n", input_number);
} |
So if input number is 27, then 27 mod 2 is 1, making that condition true in C's eyes. The same convention is used in many programming languages.
Let's translate that to Ruby.
code: | if input_number % 2
puts "#{input_number} is odd."
else
puts "#{input_number} is even."
end |
Now, if I input 27, I get the same answer, but let's try both with 42. Well, 42 mod 2 is 0, so C sees that as false and prints that the number is even.
Ruby though...
42 mod 2 is still 0 in Ruby, but it says the number is odd.
This happens because Ruby considers any value other than false or nil to be true. Zero is not automatically false. So, we have to be a bit more clear.
code: | if input_number % 2 == 1
puts "#{input_number} is odd."
else
puts "#{input_number} is even."
end |
This is an imprtant behavior to keep in mind. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
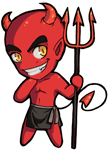
|
Posted: Sun Nov 13, 2005 4:25 pm Post subject: (No subject) |
|
|
does this have to do with the fact that 0 is an integer object? |
|
|
|
|
 |
wtd
|
Posted: Sun Nov 13, 2005 4:50 pm Post subject: (No subject) |
|
|
It has to do with the fact that zero is not false or nil.  |
|
|
|
|
 |
rizzix
|
Posted: Sun Nov 13, 2005 5:48 pm Post subject: (No subject) |
|
|
shouldn't nil be nil.. why should it be false...
false should be false.. true should be true.. everything else should be well.. NON-Boolean.. thus it should result in a syntax error... i really hate this thing about perl and C.. and to think they'd support such a thing in Ruby..
You know i really though Ruby and Haskell were at the same level of well-formedness... but apprently they are not..
(hmm but given that it's a scripting language i guess it could be excused) |
|
|
|
|
 |
wtd
|
Posted: Sun Nov 13, 2005 7:46 pm Post subject: (No subject) |
|
|
rizzix wrote: shouldn't nil be nil.. why should it be false...
false should be false.. true should be true.. everything else should be well.. NON-Boolean.. thus it should result in a syntax error... i really hate this thing about perl and C.. and to think they'd support such a thing in Ruby..
You know i really though Ruby and Haskell were at the same level of well-formedness... but apprently they are not..
(hmm but given that it's a scripting language i guess it could be excused)
Haskell and Ruby are different on many levels. Haskell is strictly-typed, lazily evaluated, and purely functional. Ruby is none of these things.
That doesn't make either of them better than the other. |
|
|
|
|
 |
rizzix
|
Posted: Sun Nov 13, 2005 10:33 pm Post subject: (No subject) |
|
|
not really... well as i stated above.. treating more than one kind of value as another kind does infact make a language worse than another.. Undefined polymorphism.. usually leads to unprecitable results.. Since it requires the programmer to be aware of more things the language does "automagically" for you, under the hood.. This is the same flaw present in C/C++.. |
|
|
|
|
 |
|
|