help with basics
Author |
Message |
TokenHerbz

|
Posted: Fri Oct 28, 2005 2:53 pm Post subject: help with basics |
|
|
ok, so wtd, i mean return 0, as in
code: |
#include <iostream.h>
int main() {
int myAge = 22;
int yourAge = 122;
cout << "My age is " << myAge << endl;
cout << "Your age is " << yourAge << endl;
return 0;
}
|
**Blah i didt test it, so dont hate me if its wrong
Is the return feature nessesary? what does it do? its purpose's, when to use it, etc:
Also i have been noticing that when i compile the programs, they do not stay open, thus i cant see my work, and cant tell if im doing things correctly.
overview..
1) Help explain the "return 0;" feature
2) Help me keep projects open longer
thanks:) |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
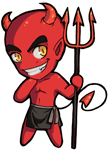
|
Posted: Fri Oct 28, 2005 3:18 pm Post subject: (No subject) |
|
|
return just ends the function, and since it's main, ends the program as well. 0 stands for a successful execution, while returning any other value will indicate various error codes for the operating system to figure out.
you don't neccessarely need it, but then you'd need to change your function name to
And you should execute your programs though a terminal / console (start -> run -> cmd ) |
|
|
|
|
 |
TokenHerbz

|
Posted: Fri Oct 28, 2005 3:21 pm Post subject: (No subject) |
|
|
EDIT::
I solved my problems! |
|
|
|
|
 |
Mazer

|
Posted: Fri Oct 28, 2005 3:52 pm Post subject: (No subject) |
|
|
You *could* say that your main function is going to return an int and end up not returning anything at all. Not that I'd recommend it.
Example:
code: | #include <iostream>
int main()
{
std::cout << "Hello, world!" << std::endl;
}
|
Compiles and executes without errors. Shocking, I know. |
|
|
|
|
 |
md

|
Posted: Fri Oct 28, 2005 4:51 pm Post subject: (No subject) |
|
|
Coutsos wrote: You *could* say that your main function is going to return an int and end up not returning anything at all. Not that I'd recommend it.
Example:
code: | #include <iostream>
int main()
{
std::cout << "Hello, world!" << std::endl;
}
|
Compiles and executes without errors. Shocking, I know.
That depends on you warning level; generally speaking it's a bad idea not to return an int in main, as is declairing main to return void, or take no arguments. Just because you can do something doesn't mean that you should. |
|
|
|
|
 |
wtd
|
Posted: Fri Oct 28, 2005 5:08 pm Post subject: (No subject) |
|
|
Coutsos wrote: Shocking, I know.
\
Not especially. The standard does dictate that if control flow hits the end of main with no return, it will return 0. |
|
|
|
|
 |
wtd
|
Posted: Fri Oct 28, 2005 5:11 pm Post subject: Re: help with basics |
|
|
code: |
#include <iostream.h>
int main() {
int myAge = 22;
int yourAge = 122;
cout << "My age is " << myAge << endl;
cout << "Your age is " << yourAge << endl;
return 0;
}
|
Bad. It will work, but "iostream.h" is deprecated. This means that future compilers do not have to support it.
Instead you should use "iostream" and make your use of the "std" (standard) namespace explicit, using either "using namespace std;" or prefixing things like cout and endl with "std::". |
|
|
|
|
 |
Mazer

|
Posted: Fri Oct 28, 2005 5:24 pm Post subject: (No subject) |
|
|
Cornflake wrote: Just because you can do something doesn't mean that you should.
Hence I did not recommend it.
wtd wrote: Not especially. The standard does dictate that if control flow hits the end of main with no return, it will return 0.
I know, we talked about it before. And I still stand by my dislike of it. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
wtd
|
Posted: Fri Oct 28, 2005 5:37 pm Post subject: (No subject) |
|
|
In addition, the zero being returned is the program's way of telling the operating system that the program completed successfully.
Anything else indicates that some kind of error occurred. The exact meaning of those values is dependent on the operating system. |
|
|
|
|
 |
|
|