Author |
Message |
md

|
Posted: Mon Oct 24, 2005 11:10 pm Post subject: Quick contest (all languages) |
|
|
Ok... so I gave the challenge on IRC, but I figure I'll open it to anyone on compsci
The Challenge: write a function to check to see if a string is a palendrome. It should only one parameter, the string, and return a boolean value of true if the string is a palendrome, or false if it is not.
[edit] You can optionally also pass a second parameter to specify the length of the string, just to reduce the overhead of recursion...
The Judges: I'll judge who wins by who can write the shortest code (instruction wise), who can write the fastest code (order n wise), and who's code is easiest to read. I might get a few other people (wtd, rizzix) to help judge too if I can.
The prize: the proze will be all my bits devided amongst the winners, or if there is a mod who will sponsor this however much they feel like giving. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
[Gandalf]

|
Posted: Mon Oct 24, 2005 11:15 pm Post subject: (No subject) |
|
|
code: | fcn checkPalindrome (word : string) : boolean
for i : 1 .. length (word) div 2
if word (i) ~= word (length (word) - i + 1) then
result false
end if
end for
result true
end checkPalindrome |
|
|
|
|
|
 |
md

|
Posted: Mon Oct 24, 2005 11:18 pm Post subject: (No subject) |
|
|
My C/C++ entry so people have an idea how one could do it
c++: |
bool Palindrome(char *string, int length)
{
return (length <= 0) || (string[0] == string[length-1]) && Palindrome(++string, length-2);
}
|
|
|
|
|
|
 |
beard0

|
Posted: Mon Oct 24, 2005 11:20 pm Post subject: (No subject) |
|
|
Beat this :)
Turing: | fcn isPalindrome (str : string) : boolean
result length(str )<= 1 or (str (1) = str (length (str )) and isPalindrome (str (2 .. length (str ) - 1)))
end isPalindrome |
Hooray for Turing short circuit logic evaluation! |
|
|
|
|
 |
beard0

|
Posted: Mon Oct 24, 2005 11:29 pm Post subject: (No subject) |
|
|
By the way Cornflake's "idea how one could do it" doesn't actually work, you need to expand on it. |
|
|
|
|
 |
md

|
Posted: Mon Oct 24, 2005 11:31 pm Post subject: (No subject) |
|
|
Yes, yes... so my mind was a little slow after my exam... it's changed and it does now work, I've tested it.
[edit] arg... it's harder then it seems to write it on one line... see my sig
[edit 2] my god I'm dumb... but it now works... tested verilly... |
|
|
|
|
 |
wtd
|
Posted: Tue Oct 25, 2005 12:14 am Post subject: (No subject) |
|
|
O'Caml:
code: | let rec palindrome str =
let len = String.length str in
if len <= 1 then true
else if str.[0] = str.[len - 1] then
palindrome (String.sub str 1 (len - 2))
else false |
|
|
|
|
|
 |
Martin

|
Posted: Tue Oct 25, 2005 12:18 am Post subject: (No subject) |
|
|
For an added challenge:
Do it as a one liner recursive call to main in C, returning a number other than 0 if argv[0] is a palindrome, 0 if it isn't.
code: | int main (int argc; char**argv) {
return <yourcodehere>;
} |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
wtd
|
Posted: Tue Oct 25, 2005 12:42 am Post subject: (No subject) |
|
|
Martin wrote: For an added challenge:
Do it as a one liner recursive call to main in C, returning a number other than 0 if argv[0] is a palindrome, 0 if it isn't.
code: | int main (int argc; char**argv) {
return <yourcodehere>;
} |
Did you actually mean argv[0]? That's typically the executable name. |
|
|
|
|
 |
Martin

|
Posted: Tue Oct 25, 2005 1:15 am Post subject: (No subject) |
|
|
Sorry, yeah, I forgot about that. It's been a while since I've used C. argv[1]; |
|
|
|
|
 |
TokenHerbz

|
Posted: Tue Oct 25, 2005 2:02 am Post subject: (No subject) |
|
|
To: Cornflake
you said you'd gimmy 5 bits on IRC for trying
When can i expect them? |
|
|
|
|
 |
wtd
|
Posted: Tue Oct 25, 2005 3:10 am Post subject: (No subject) |
|
|
Ruby:
code: | class String
def is_palindrome?
if length <= 1
true
elsif self[0] == self[-1]
self[1..-2].is_palindrome?
else
false
end
end
end |
|
|
|
|
|
 |
Tony
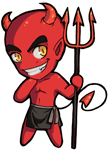
|
Posted: Tue Oct 25, 2005 7:41 am Post subject: (No subject) |
|
|
Ruby: |
class String
def is_palindrome?
self == self.reverse
end
end
|
 |
|
|
|
|
 |
md

|
Posted: Wed Oct 26, 2005 10:51 am Post subject: (No subject) |
|
|
Methinks I'll leave this open until next weekend and then I'll judge winners... and martin; I've almost figured out how to beat your challenge  |
|
|
|
|
 |
goomba
|
Posted: Wed Oct 26, 2005 5:56 pm Post subject: (No subject) |
|
|
Python for the win!
code: |
def isPalindrome(word):
if word[::-1] in word:
return True
return False
|
|
|
|
|
|
 |
|