GUI Rock, Paper, Scissor's Game
Author |
Message |
Planet_Fall

|
Posted: Sun Jan 26, 2014 10:06 pm Post subject: GUI Rock, Paper, Scissor's Game |
|
|
My first GUI game, has buttons and a score keeper. Enjoy!
Turing: |
% Rock, Papper, Siccors
% Planet_Fall
% Build Window
import GUI
View.Set ("graphics:300;150")
GUI.SetBackgroundColor (white)
% Declare Types
type choice :
record
ROCK : boolean
PAPPER : boolean
SCISSORS : boolean
end record
% Declare Variables
var opponnetchoice : choice
var picker, counter_refresh : int
var win, lose, draw : real := 0
% Set to Default
opponnetchoice.ROCK := false
opponnetchoice.SCISSORS := false
opponnetchoice.PAPPER := false
counter_refresh := 0
% Procedures
procedure showscore
cls
put skip
put "WINS : " : 10, win : 3
put "DRAWS : " : 10, draw : 3
put "LOSES : " : 10, lose : 3
var total : real := (lose + draw + win )
put "TOTAL : " : 10, total : 3
put skip
end showscore
procedure opponnetpick
picker := Rand.Int (1, 3)
if picker = 1 then
opponnetchoice.ROCK := true
elsif picker = 2 then
opponnetchoice.SCISSORS := true
elsif picker = 3 then
opponnetchoice.PAPPER := true
end if
end opponnetpick
procedure Rock
opponnetpick
if opponnetchoice.PAPPER = true then
put "YOU HAVE LOST"
lose + = 1
opponnetchoice.PAPPER := false
counter_refresh + = 1
if counter_refresh = 5 then
delay (500)
cls
GUI.Refresh
counter_refresh := 0
end if
elsif opponnetchoice.ROCK = true then
put "YOU HAVE DRAWED"
opponnetchoice.ROCK := false
counter_refresh + = 1
draw + = 1
if counter_refresh = 5 then
delay (500)
cls
GUI.Refresh
counter_refresh := 0
end if
elsif opponnetchoice.SCISSORS = true then
put "YOU HAVE WON"
opponnetchoice.SCISSORS := false
counter_refresh + = 1
win + = 1
if counter_refresh = 5 then
delay (500)
cls
GUI.Refresh
counter_refresh := 0
end if
end if
end Rock
procedure Papper
opponnetpick
if opponnetchoice.PAPPER = true then
put "YOU HAVE DRAWED"
opponnetchoice.PAPPER := false
counter_refresh + = 1
draw + = 1
if counter_refresh = 5 then
delay (500)
cls
GUI.Refresh
counter_refresh := 0
end if
elsif opponnetchoice.ROCK = true then
put "YOU HAVE WON"
opponnetchoice.ROCK := false
counter_refresh + = 1
win + = 1
if counter_refresh = 5 then
delay (500)
cls
GUI.Refresh
counter_refresh := 0
end if
elsif opponnetchoice.SCISSORS = true then
put "YOU HAVE LOST"
opponnetchoice.SCISSORS := false
counter_refresh + = 1
lose + = 1
if counter_refresh = 5 then
delay (500)
cls
GUI.Refresh
counter_refresh := 0
end if
end if
end Papper
procedure Scissors
opponnetpick
if opponnetchoice.PAPPER = true then
put "YOU HAVE WON"
opponnetchoice.PAPPER := false
counter_refresh + = 1
win + = 1
if counter_refresh = 5 then
delay (500)
cls
GUI.Refresh
counter_refresh := 0
end if
elsif opponnetchoice.ROCK = true then
put "YOU HAVE LOST"
opponnetchoice.ROCK := false
counter_refresh + = 1
lose + = 1
if counter_refresh = 5 then
delay (500)
cls
GUI.Refresh
counter_refresh := 0
end if
elsif opponnetchoice.SCISSORS = true then
put "YOU HAVE DRAWED"
opponnetchoice.SCISSORS := false
counter_refresh + = 1
draw + = 1
if counter_refresh = 5 then
delay (500)
cls
GUI.Refresh
counter_refresh := 0
end if
end if
end Scissors
% Build Buttons
var a : int := GUI.CreateButton (10, 35, 0, "ROCK", Rock )
var b : int := GUI.CreateButton (100, 35, 0, "PAPPER", Papper )
var c : int := GUI.CreateButton (200, 35, 0, "SCISSORS", Scissors )
var d : int := GUI.CreateButton (110, 5, 0, "EXIT", showscore )
% Button Press
loop
exit when GUI.ProcessEvent
end loop
|
P.S
I used Turing 4.1.1 |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
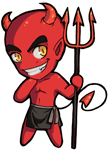
|
Posted: Mon Jan 27, 2014 12:19 am Post subject: RE:GUI Rock, Paper, Scissor\'s Game |
|
|
code: |
if counter_refresh = 5 then
delay (500)
cls
GUI.Refresh
counter_refresh := 0
end if
|
is the same every time -- you could have just one copy of this code at the end of the procedure, instead of having the same code for every possibility.
Similarly, all 3 procedures have identical structure. You can collapse them down into a single procedure that takes an argument for the user choice. Your GUI declarations would look something like
code: |
var a : int := GUI.CreateButton (10, 35, 0, "ROCK", make_a_choice(choice.ROCK))
...
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Planet_Fall

|
Posted: Mon Jan 27, 2014 10:55 am Post subject: Re: GUI Rock, Paper, Scissor's Game |
|
|
I'm new to GUI so that last example, goes a bit over my head. Do you know of a good GUI tutorial? And if it's possible could you run me through the last example? |
|
|
|
|
 |
Zren

|
Posted: Mon Jan 27, 2014 7:35 pm Post subject: Re: RE:GUI Rock, Paper, Scissor\'s Game |
|
|
Why use 3 different booleans to represent 1 thing that can have 3 different states? Each of those booleans have 2 different states. 3 booleans have a sum of 8 (2*2*2) different states.
code: |
0 0 0
0 0 1
0 1 0
0 1 1
1 0 0
1 0 1
1 1 0
1 1 1
|
Instead, try using a single integer that can represent way more than 3 different states. Map certain values to a particular state. Eg: ROCK = 1, PAPER = 2, SCISSORS = 3
You can then do if choice = ROCK then ... which reads off better as well.
Tony @ Mon Jan 27, 2014 12:19 am wrote:
Similarly, all 3 procedures have identical structure. You can collapse them down into a single procedure that takes an argument for the user choice. Your GUI declarations would look something like
code: |
var a : int := GUI.CreateButton (10, 35, 0, "ROCK", make_a_choice(choice.ROCK))
...
|
Fairly certain you can only use procedures without any arguments when using the GUI module. That doesn't stop you from creating a proc for when rock is pressed, that calls another function like so.
Turing: |
var ROCK : int := 1
proc makeChoice (choice : int)
% ...
end makeChoice
proc onRockButtonPress ()
makeChoice (ROCK )
end onRockButtonPress
var rockButton : int := GUI.CreateButton (10, 35, 0, "ROCK", onRockButtonPress )
|
|
|
|
|
|
 |
|
|