Plug-ins
Author |
Message |
Cervantes

|
Posted: Sun Apr 02, 2006 4:02 pm Post subject: Plug-ins |
|
|
Has anyone ever programmed anything that allows users to write plug-ins? Or, does anyone know how such could be accomplished?
Thus far, my thoughts on the subject are sketchy. I'm thinking of each plug-in being a module that defines a "run" method. Each plug-in's run method is called, and that's that. The general integrity of the program would be maintained because most of the data is hidden within objects. However, I'm wondering whether too much is hidden within objects, and whether the plug-ins would actually have some power/flexibility. [This is for Rubidium, by the way.]
Thanks |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
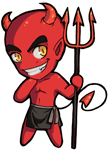
|
Posted: Sun Apr 02, 2006 4:39 pm Post subject: (No subject) |
|
|
if this is in Ruby, can't you allow for mixins? where each plugin ads something to existing classes within the program |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Cervantes

|
Posted: Sun Apr 02, 2006 5:54 pm Post subject: (No subject) |
|
|
That's a good idea, Tony. But I think there's still more.
Say I want to make a plug-in for logging. How I'm currently doing logging is like this: The Server class runs a thread to listen for input from the given server. Among other things, this thread parses the input and sends lines out to the appropriate Channel objects. The Channel objects write lines to their appropriate log file.
A plug-in for logging would need to define a bunch of stuff for the Channel class, but it would also need to modify that thread in the Server class a little bit. How might that be done? Maybe modifying the thread isn't necessary. Perhaps the thread could already have the code to send the lines out to the channel, but that means that some plugins would require the base code to do something. This reduces the flexibility of the plug-ins. Although if the base code is robust enough that most plug-ins can exist like this, then the few that can't might exist by defining their own threads to mix in to the appropriate classes.
I could see how a single plug-in could modify more than one class. Each plug-in could be a module that contains one or more modules. Each of these submodules is mixed into a given class.
Thanks again  |
|
|
|
|
 |
Cervantes

|
Posted: Tue Apr 11, 2006 8:56 pm Post subject: (No subject) |
|
|
Why are we worrying about mixins when we could just extend the classes themselves? Thanks cartoon_shark.
Currently I'm thinking that if a method needs to be slightly redefined, this will be done by aliasing the original definition of the method to a new name then redefining it, using the old definition of the method where necessary. The old definition of the method can then be undefed to prevent the class from becoming too cluttered.
Maybe this process could be simplified by making all classes for plugins inherit from a RubidiumPlugin class. I'll look into it more, but it might be possible to overwrite the code to create a new method. If so, I could redefine it to add automatic aliasing and undefing.
Seeing as this thread is now totally Ruby oriented, moved to [Ruby Help] |
|
|
|
|
 |
Cervantes

|
Posted: Wed Jul 19, 2006 6:52 pm Post subject: (No subject) |
|
|
I should specify my solution to this problem. I should have done this a few months ago.
I did indeed chose to let plugins extend the base classes of Rubidium, themselves. This has its ups and downs. One of the biggest 'ups' is that it required no additional work on my part, aside from a little bit of code to recursively `load' all .rb files in the plugins folder.
But, what about extending methods, rather than completely overwriting them?
I want to be able to do something like this:
code: |
class Foo
def bar
puts "baz"
end
end
# Reopen Foo class and extend the bar method
class Foo
def bar
# call old definition of bar
puts "bar method extended. bar method now also says, "
puts "qux."
end
end
Foo.new.bar
# Hoped output as follows:
# baz
# bar method extended. bar method now also says,
# qux.
|
This behaviour should immediately spring one idea to mind: super!
So I could make a new class that inherits from the base class, use super where necessary to extend the method I want, and then...
But the trouble is that the main program doesn't know to use this new child class. How can we get around this?
We can overwrite the constant for the original base class with the constant for the new child class.
code: |
class Foo
def bar
puts "baz"
end
end
class FooMod < Foo
def bar
super
puts "bar method extended. bar method now also says, "
puts "qux."
end
end
# Remove the constant from existance
Object.instance_eval {remove_const :Foo}
# Assign the FooMod constant to our "new" Foo constant
Foo = FooMod
# Try it!
Foo.new.bar
# Output is as hoped!
# Output is the following:
# baz
# bar method extended. bar method now also says,
# qux.
|
|
|
|
|
|
 |
Cervantes

|
Posted: Wed Jul 19, 2006 7:03 pm Post subject: (No subject) |
|
|
I should also add that in Ruby, constants are not really constant. I mean, if we can remove it from existance and then rewrite it, it's not constant.
But we don't even have to do that much work.
code: |
# In the reign of Douglas Adams...
Universe = 42
# Some pesky non-believer comes along...
Universe = -3
puts Universe
|
output wrote:
foo.rb:5: warning: already initialized constant Universe
-3
So ruby gave us a warning, but it still gave the constant a new value. Is Ruby dynamic or what?
The reason I went through that business of removing the constant is simply to avoid getting that warning. The other alternative would be to run my program with the -W0 (dash w zero, meaning warning level is set to zero -- silence) option, but that isn't very professional. |
|
|
|
|
 |
|
|