Reading Specific Data in Files
Author |
Message |
NewHydrolisk
|
Posted: Fri Jul 02, 2010 4:58 pm Post subject: Reading Specific Data in Files |
|
|
Hello there. I am trying to create save and load functions that will write data to a file and read data from a file respectively. I am using Dev-C++, so my compiler would be MinGW. I think.
Specifically, the problem I have at hand is the data reading from the file. I have solved the saving portion of my task.
code: | void save() // game save
{
ofstream charFile( "charFile.sav", ios::trunc | ios::binary ); // open output file stream for "charFile"
if( charFile.is_open() == 1 )
{
charFile << " destiny" << endl; // line 1
charFile << destiny << endl; // line 2
charFile << " level" << endl; // l 3
charFile << LVL << endl; // l 4
charFile << " experience" << endl; // l5
charFile << EXP << endl; // l 6
charFile << " hitpoints" << endl; // l 7
charFile << HP << endl; // l 8
charFile << " attack" << endl; // l 9
charFile << ATK << endl; // l 10
charFile << " defence" << endl; // l 11
charFile << DEF << endl; // l 12
// l 13
charFile.close();
}
else
{
error( "Could not save game." );
}
}
void load() // game load
{
ifstream charFile( "charFile.sav", ios::binary ); // open input fstream for "charFile"
if( charFile.is_open() == 1 )
{
}
else
{
error( "Could not load game. Previous game may not have been saved." );
}
} |
As you can see, the written binary file would have a total of 13 lines, with every line stating a comment or the comment's value. The saving portion works fine.
However, I am entirely at a loss as to how I would approach "loading" the same file into the program.
I know that I only need to load every even-numbered line from 2 to 12 and assign the appropriate variables the read values, but I simply do not know how to go about doing so.
-
If I do anything/say anything stupid, I'll try to fix it if you tell me. I've very little practical C++ experience, I'm still learning C++, and I'm more or less new to the forum. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
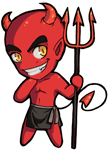
|
Posted: Sun Jul 04, 2010 12:54 pm Post subject: RE:Reading Specific Data in Files |
|
|
You are opening the files for binary input/output, but your code suggests that you actually want to be in the text mode (there are no "2nd line" in binary mode, there's only "42 bytes in, here's a stream of bits").
http://www.cplusplus.com/doc/tutorial/files/ |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
NewHydrolisk
|
Posted: Sun Jul 04, 2010 2:42 pm Post subject: Re: Reading Specific Data in Files |
|
|
oops okay I got/changed it now.
c++: | void load() // game load
{
ifstream charFile( "charFile.sav" ); // open input fstream for "charFile"
if( charFile.is_open() == 1 )
{
string line;
for( int i = 0; i != 13; i++ )
{
getline( charFile, line );
if( i % 2 == 1 )
{
cout << line << endl;
}
}
charFile.close();
}
else
{
error( "Could not load game. Previous game may not have been saved." );
}
} |
Here's what I have now. It works perfectly as intended. However, I'm just curious if there is a more efficient way of reading the file, since the program would read the unnecessary header lines too.
I don't think I can use seekg() because the odd lines carry values that might vary in number of digits. |
|
|
|
|
 |
|
|