Collision Detection Problem
Author |
Message |
Monduman11

|
Posted: Mon May 17, 2010 3:27 pm Post subject: Collision Detection Problem |
|
|
What is it you are trying to achieve?
make my character not fall off the map
What is the problem you are having?
keeps on falling off in places where collision should work
Describe what you have tried to solve this problem
code, making the black boxes bigger
Post any relevant code (You may choose to attach the file instead of posting the code if it is too long)
In the download. everything is there for the game to run and work.
Turing: |
<Add your code here>
|
Please specify what version of Turing you are using
4.1.1
Description: |
Everything is in the folder |
|
 Download |
Filename: |
New Folder.rar |
Filesize: |
64.8 KB |
Downloaded: |
94 Time(s) |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Monduman11

|
Posted: Mon May 17, 2010 6:17 pm Post subject: Re: Collision Detection Problem |
|
|
im sorry if i didn't fully explain my problem, but whats actually wrong with the game is that it sometimes just out of the blue the collision detection stop working and instead of my character stopping at the black it just goes through it. ive tried doing everything i can think of. ive tried making the black boxes in the background image be bigger but it doesnt seem to have an effect. ive even asked my cs teacher if she can help me and she just said she doesnt know whats wrong with it. i would greatly appreciate it if someone could look at the code and explain to me why its not working properly sometimes. im not asking for anyone to write me the code just for someone to hint at what im doing wrong.
i will be attaching the part with the collision detection below this.
|
|
|
|
|
 |
Tony
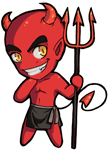
|
Posted: Mon May 17, 2010 6:49 pm Post subject: RE:Collision Detection Problem |
|
|
You need to have a better idea of where exactly you are checking for colours, and what is at those spots, when you think your collision has failed. More so since the distance at which you make a check for a single point is not even the same as the distance by which the character moves (maybe, I have no idea what variable 'z' is).
Try drawing some visual cues.
code: |
Draw.Oval(posx - 10, posy + 24, 2, 2, red)
Draw.Oval(posx + 10, posy + 24, 2, 2, green)
if chars (KEY_LEFT_ARROW) and whatdotcolour (posx - 10, posy + 24) not= black then
sprite := pic1
z := z + 17
elsif chars (KEY_RIGHT_ARROW) and whatdotcolour (posx + 10, posy + 24) not= black then
sprite := pic
z := z - 17
else
velx := 0
end if
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Monduman11

|
Posted: Mon May 17, 2010 7:00 pm Post subject: Re: RE:Collision Detection Problem |
|
|
Tony @ Mon May 17, 2010 6:49 pm wrote: You need to have a better idea of where exactly you are checking for colours, and what is at those spots, when you think your collision has failed. More so since the distance at which you make a check for a single point is not even the same as the distance by which the character moves (maybe, I have no idea what variable 'z' is).
Try drawing some visual cues.
code: |
Draw.Oval(posx - 10, posy + 24, 2, 2, red)
Draw.Oval(posx + 10, posy + 24, 2, 2, green)
if chars (KEY_LEFT_ARROW) and whatdotcolour (posx - 10, posy + 24) not= black then
sprite := pic1
z := z + 17
elsif chars (KEY_RIGHT_ARROW) and whatdotcolour (posx + 10, posy + 24) not= black then
sprite := pic
z := z - 17
else
velx := 0
end if
|
oh srry the 'z' is the variable that i used for the sidescrolling. so when i press the right or left button the z changes. i should change the variable lol, but i dont really get whats with the draw oval...?? how could i improve the collision detection though? cause ive only been doing this for about 1 month and the only way ive learned to detect collision is with the whatdotcolour and the box one, but i dont know how to do the box one properly plus it would mean id have to do it for the whole game which would take a long time.
|
|
|
|
|
 |
Tony
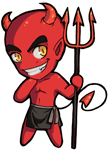
|
Posted: Mon May 17, 2010 7:14 pm Post subject: RE:Collision Detection Problem |
|
|
The most common problems is that people don't check for collision in the right spots, or the colour checked against is not what is expected (draw order matters, visual effects mess with checks, etc.)
Drawing outlined ovals will remind you where you are actually checking for collision, and will help you debug as to what is going on.
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Monduman11

|
Posted: Mon May 17, 2010 7:18 pm Post subject: Re: RE:Collision Detection Problem |
|
|
Tony @ Mon May 17, 2010 7:14 pm wrote: The most common problems is that people don't check for collision in the right spots, or the colour checked against is not what is expected (draw order matters, visual effects mess with checks, etc.)
Drawing outlined ovals will remind you where you are actually checking for collision, and will help you debug as to what is going on.
ah the only problem is that im horrible at debugging cause i havent really had any experience with it. especially when it comes to debugging a game. maybe if my teacher wasnt so horrible id have learned something, instead all she does is assign the work and she chills while we try to figure stuff out... and when u ask for help all she does it say she doesnt know and walk away.
|
|
|
|
|
 |
Insectoid

|
Posted: Mon May 17, 2010 7:26 pm Post subject: RE:Collision Detection Problem |
|
|
You'd better get good at debugging quick. "Put" is your friend here -output variables at different stages and see if they are what you expect them to be. If they aren't, backtrack up to where that variable changes and check it there. Keep backtracking until you hit a spot where the variable is what it's supposed to be. That's where your problem is.
|
|
|
|
|
 |
Monduman11

|
Posted: Mon May 17, 2010 7:28 pm Post subject: Re: RE:Collision Detection Problem |
|
|
Insectoid @ Mon May 17, 2010 7:26 pm wrote: You'd better get good at debugging quick. "Put" is your friend here -output variables at different stages and see if they are what you expect them to be. If they aren't, backtrack up to where that variable changes and check it there. Keep backtracking until you hit a spot where the variable is what it's supposed to be. That's where your problem is.
kk ill try that... what should i be outputting though? just numbers? like put x or z? stuff like that? or more detailed? like put gravity or i dont know what else lol
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
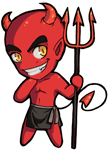
|
Posted: Mon May 17, 2010 7:31 pm Post subject: RE:Collision Detection Problem |
|
|
Well then this is an excellent opportunity to start. Programming is one of the activities where you learn best through practice.
Sometimes you need to make mistakes, to have something to work through. Having a teacher tell you to "change this line" will not help you with acquiring the ability to figure out which line was the problem.
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Insectoid

|
Posted: Mon May 17, 2010 7:33 pm Post subject: RE:Collision Detection Problem |
|
|
Any variable you think might be causing the problem. Even 'put "bleh"' can be useful (to check if a loop is running or a conditional is passed, etc). It takes practice to get good at it.
EDIT: Damnit Tony, you beat me!
|
|
|
|
|
 |
Monduman11

|
Posted: Tue May 18, 2010 2:22 pm Post subject: Re: Collision Detection Problem |
|
|
its ok i fixed it... i just changed the code a bit and made the black boxes in the background bigger and now it works fine. thx for ur help though
|
|
|
|
|
 |
|
|